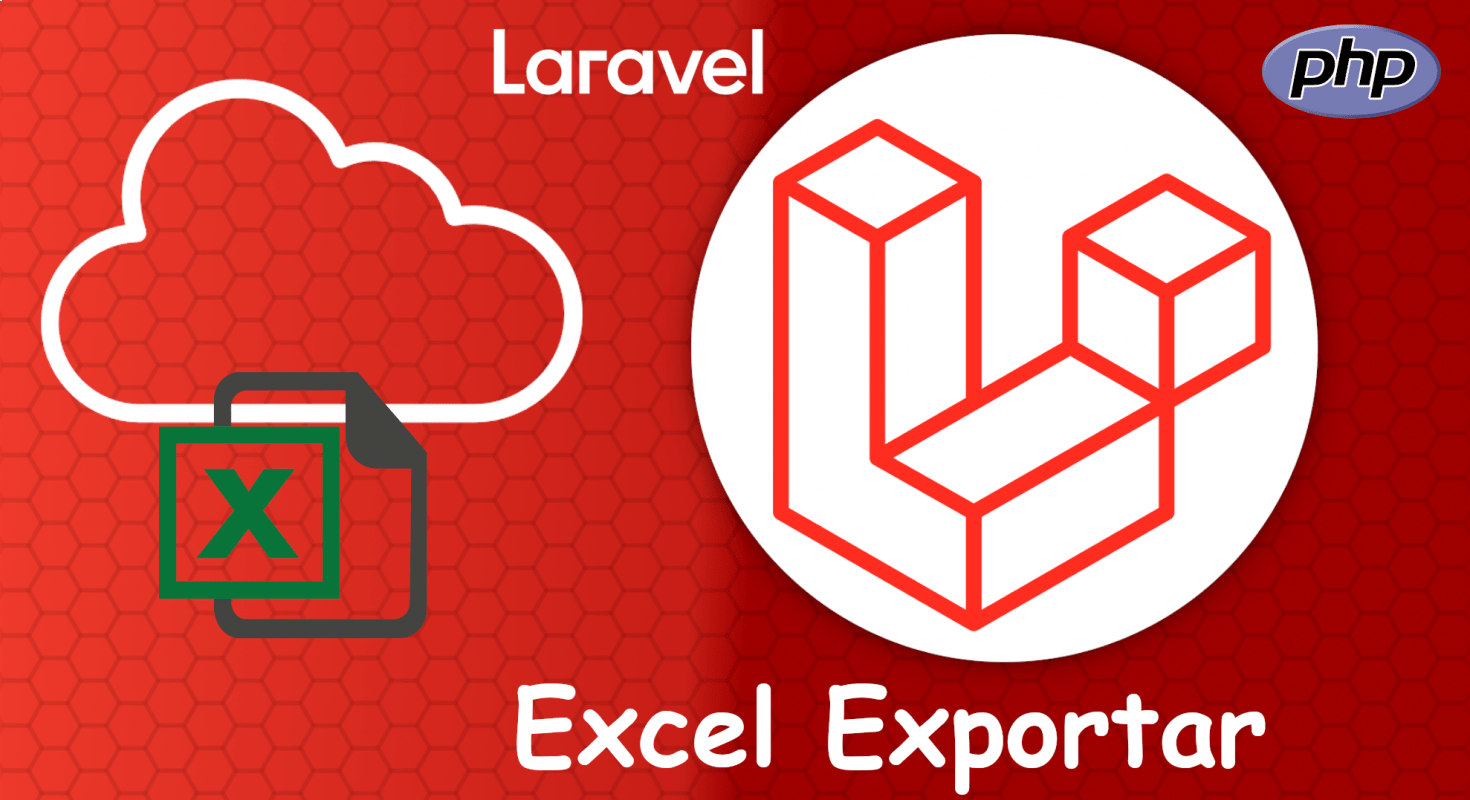
In this post we are going to see how we can generate an Excel file using Laravel, for this we are going to use a package that will greatly facilitate this entire process.
Excel files are a popular format for sharing data, if we have a list of data in Laravel, it is often necessary to export the data in an excel sheet so that it is easily consumable and can perform additional operations outside the application in Laravel, or to make reports from time to time etc... their scope is unlimited and there are many scenarios where they are very useful.
Excel files are proprietary to Microsoft Office. These files allow you to create, edit and analyze data and are presented as workbooks that contain several sheets, and are made up of cells.
In this post, we will see how to save excel files in Laravel.
Prepare the Project
For the project, we are going to use any installation that you have on Laravel, for example, the one that we discussed earlier in which we took the first steps with Laravel.
So just create a new project or the one you're doing some testing with and go ahead.
Install Laravel-Excel package
To install the package we simply have to execute the following composer command in our Laravel project:
composer require maatwebsite/excel
Laravel Excel base configurations
For the rest we don't have to do much, since like any package we have practically everything for free and we can very easily generate files in Excel format given a model to indicate the query with which we want to work.
And now, we have to create or publish the configurations that come in the default package:
php artisan vendor:publish
This will generate a configuration file that you will be able to see in the project configurations folder that we will not address in this entry since we do not need to change anything in it.
Create our export class
Once our package is installed and configured, the next thing we have to do is generate a special file from this library in which we have to implement a class with the Eloquent query (export file) on the model whose data we want to export; for this the following artisan command:
php artisan make:export PostsExport --model=Post
By passing the model option, as you can suppose, it allows us to specify the model that we want to make exportable, the data that we are going to export via Excel and in this way some methods and procedures are autogenerated when generating the file that we are going to explain below.
Our model looks like the following:
<?php
namespace App;
use App\Category;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $fillable = ['title', 'url_clean', 'content', 'category_id', 'posted'];
public function category()
{
return $this->belongsTo(Category::class);
}
}
And the category:
<?php
namespace App;
use App\Post;
use Illuminate\Database\Eloquent\Model;
class Category extends Model
{
protected $fillable = ['title', 'url_clean'];
public function post()
{
return $this->hasMany(Post::class);
}
}
This class, which is basically what will be generated on app/Export, will allow us to indicate the collection, which is basically the SQL query through our Eloquent ORM; for example, if we want to get all the records, we simply use the all() function:
use Maatwebsite\Excel\Facades\Excel;
namespace App\Exports;
use App\Post;
use Maatwebsite\Excel\Concerns\FromCollection;
class PostsExport implements FromCollection
{
/**
* @return \Illuminate\Support\Collection
*/
public function collection()
{
return Post::all();
}
}
As you can see, we simply use any model with which we want to work, which in this case is one called Post; In our export file we indicate the Eloquent operations to indicate what data we want to work with, in this case it would be with all the posts, therefore we indicate an all in the collection method, but you can place a filter with a where or something for the style to indicate a subset of data.
Exporting the Excel from the controller
Now with the previously generated class, the next thing we have to do is use it, for that from our controller or create one as you want, as we did in the Creating a form and sending Post requests entry, we create the corresponding function and use Facade Excel, we indicate the download function which receives two parameters, the previous class, as data source, and the name of the file, we obviously have to return this since it has been the response that this function will return via http, the download of a file:
public function export(){
return Excel::download(new PostsExport, 'posts.xlsx');
}
Configuring the path to export Excel
From here are the routine steps, in any process that we want to do in Laravel, in this case it would be to generate a common route that we already discussed previously:
And if we go to the route that we configured previously:
Route::get('/dashboard/excel/post-export', 'dashboard\PostController@export')->name('post.export');
You will see that we automatically download an Excel file like the following:
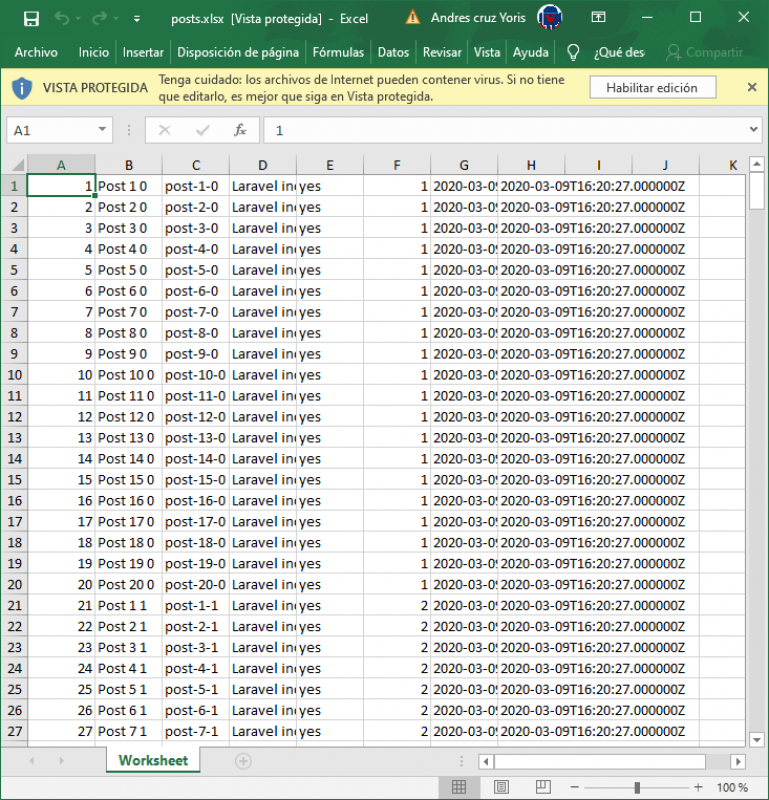
In this way, we can easily generate an excel file in Laravel; as you can see, you can apply any type of condition in the FromCollection class to avoid working with all the data pull.

Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter