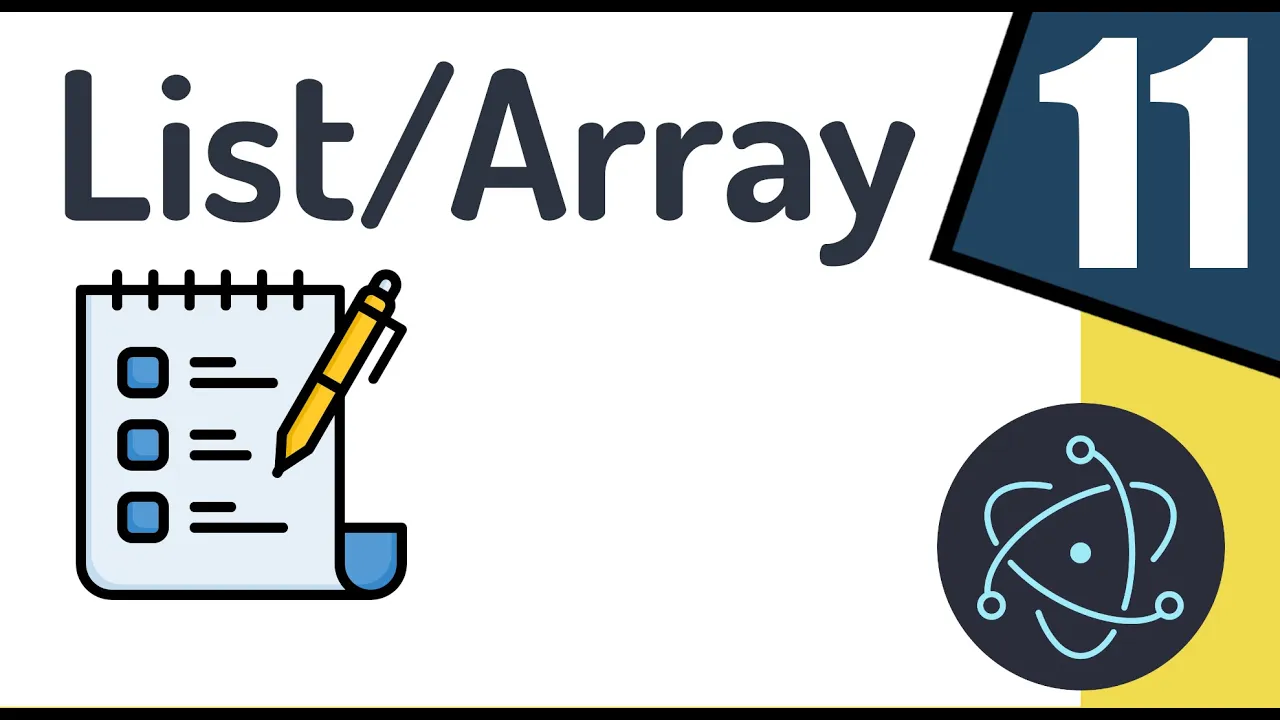
Previously we saw how to create the text box; In this section, we are going to build the list of contacts programmatically using JavaScript; for that, we will start from an array of objects with the following format:
index.html
<script>
function createContacts() {
var contacts = [
{
'name': 'Alex Alexis',
'image': 'https://randomuser.me/api/portraits/women/56.jpg',
'last_chat': [
{
'date': '9:15 AM',
'message': 'Lorem ipsum dolor sit amet consectetur adipisicing elit',
}
]
},
{
'name': 'Ramon Reed',
'image': 'https://randomuser.me/api/portraits/women/59.jpg',
'last_chat': [
{
'date': '9:15 AM',
'message': 'Lorem Hello!',
}
]
},
{
'name': 'Eli Barrett',
'image': 'https://randomuser.me/api/portraits/women/58.jpg',
'last_chat': [
{
'date': '8:55 PM',
'message': 'Lorem ipsum dolor sit ...',
}
]
},
]
}
</script>
Now, with the previous format, we are going to iterate the contacts array and build an HTML with the previous structure:
<script>
function createContacts() {
var contacts = [
***
]
var lis = ''
contacts.forEach((c) => {
lis += `<li class="p-2 card mt-2">
<div class="card-body">
<div class="d-flex">
<div>
<img class="rounded-pill me-3" width="60"
src="${c.image}">
</div>
<div>
<p class="fw-bold mb-0 text-light">${c.name}</p>
<p class="small text-muted">${c.last_chat[0]['message']}</p>
</div>
<div>
<p class="small text-muted">${c.last_chat[0]['date']}</p>
<span class="badge bg-danger rounded-pill float-end">1</span>
</div>
</div>
</div>
</li>`
})
document.querySelector('.contact').innerHTML = lis;
}
createContacts()
</script>
Remember that the previous material is part of mi curso completo sobre Electron.js
I agree to receive announcements of interest about this Blog.
In this section, we are going to build the list of contacts programmatically using JavaScript.
- Andrés Cruz